Gallery
Components
The Gallery is made up of the main Gallery
component and two optional components.
- Gallery - the main component for the images
- GalleryCard - this is an instance of the
Card
component with prebuilt margins.
id string
You can pass in an ID to the component if you need to reference it later. If you do not pass an ID, there will be a unique id added to the component.
images Array.<String>
[]
All of of the images for the gallery.
grid string
1x1
All the grid size for the gallery like 1x1, 2x2, 3x3, etc..
page integer
1
The page to start with, defaults to 1
overlay_buttons array.<Button>
Buttons that will show up when an image from the gallery is clicked.
new_image array.<Button>
A function that can be added which will add a section on the last gallery image that will let the user add a new image.
The rest of these properties work the the header of the Gallery
, so it's advised to wrap the Gallery in the GalleryCard
if you're using these.
title String
Gallery
The title to add to the Gallery
pagination boolean
true
If you want pagination to be enabled. If there are more images than your grid, this will add pagination as buttons in the header.
buttons Array.<Button>
[]
Button to add the the header in the gallery. These will be added next to the pagination buttons if pagination is enabled.
Images
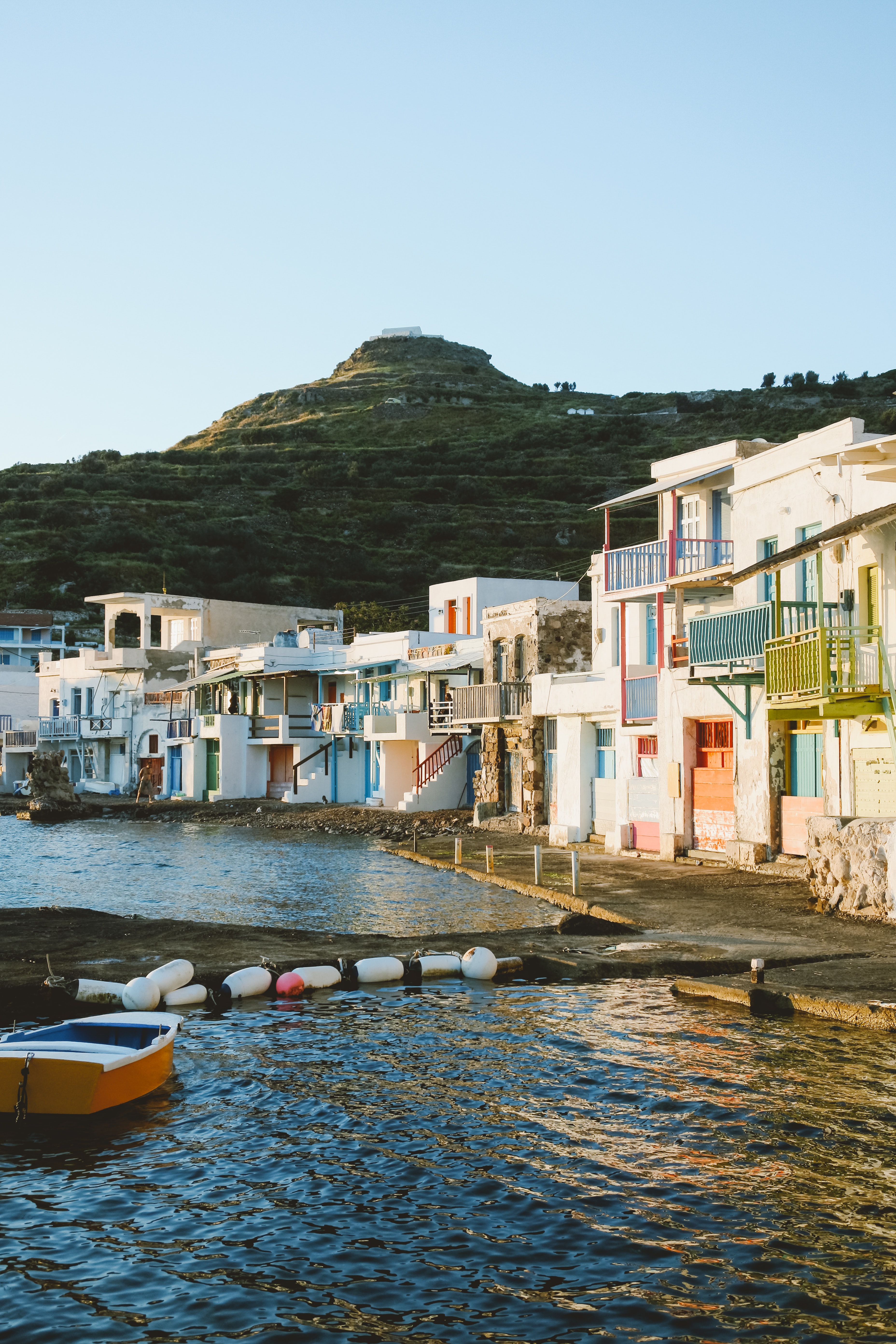
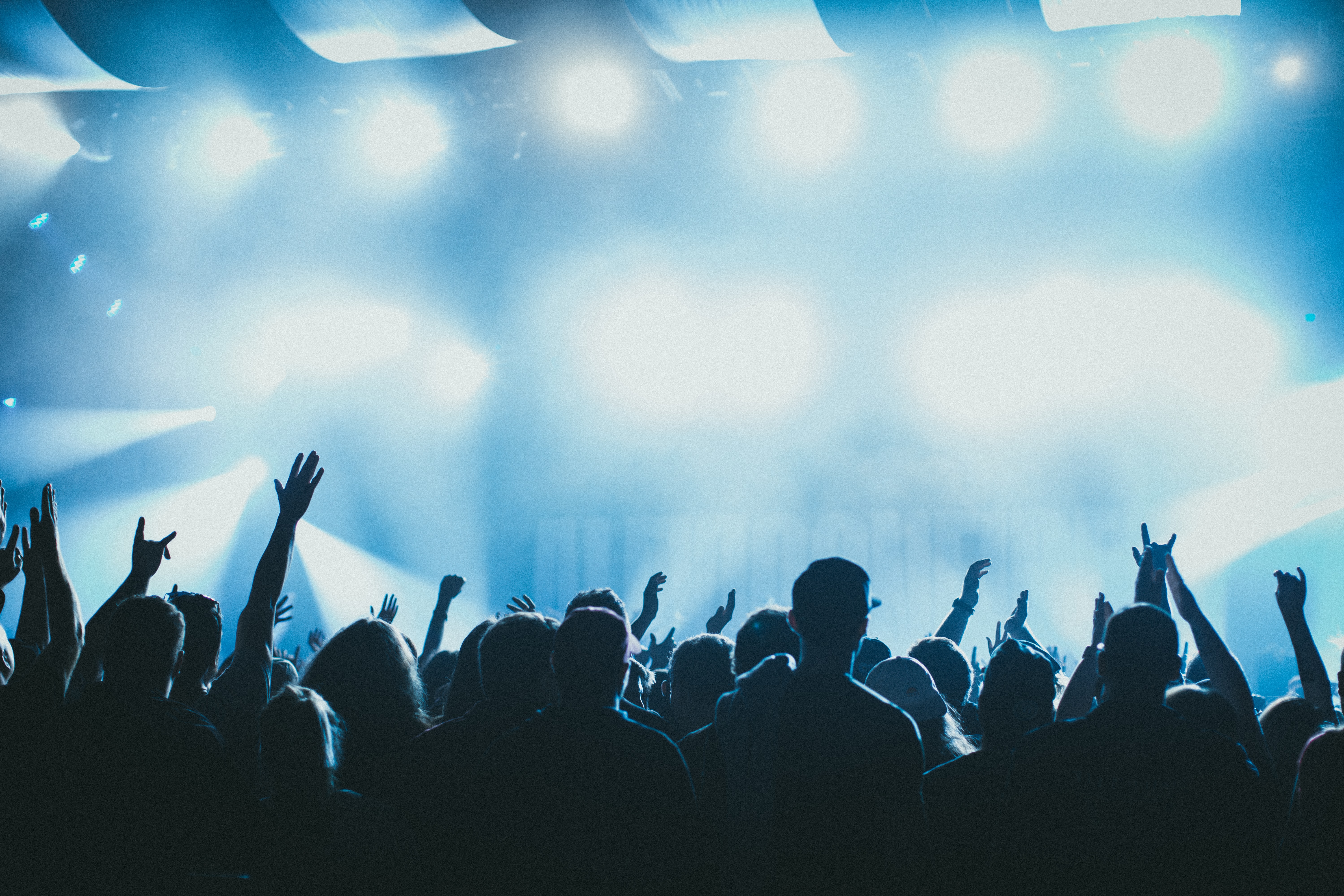
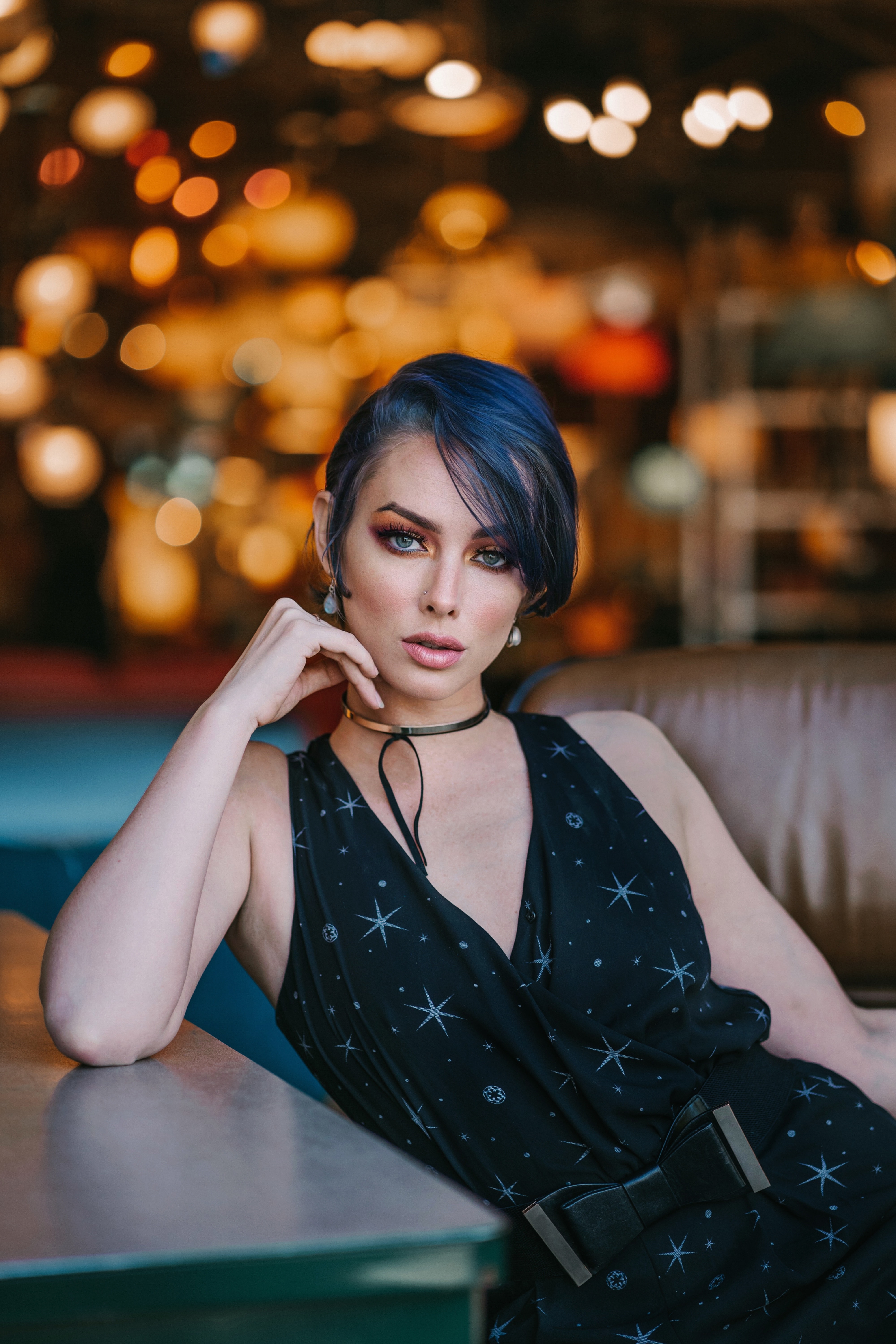
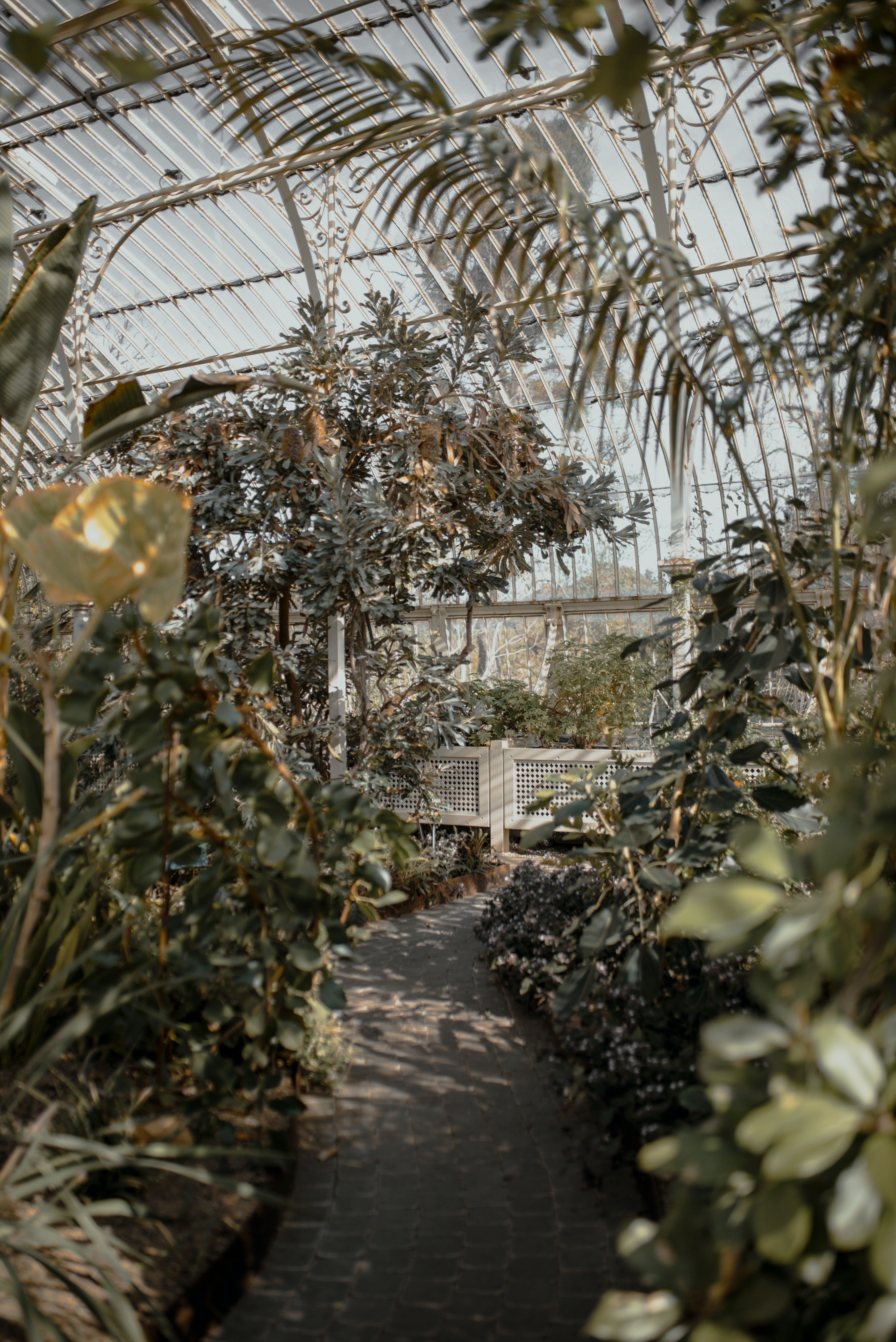
<script>
import { Gallery, GalleryCard, showModal, getFormData, showLoading, hideLoading } from "$lib/index";
import image1 from "$docs/icons/gallery/1.jpg";
import image2 from "$docs/icons/gallery/2.jpg";
import image3 from "$docs/icons/gallery/3.jpg";
import image4 from "$docs/icons/gallery/4.jpg";
import image5 from "$docs/icons/gallery/5.jpg";
import image6 from "$docs/icons/gallery/6.jpg";
import image7 from "$docs/icons/gallery/7.jpg";
import image8 from "$docs/icons/gallery/8.jpg";
let images = [image1, image2, image3, image4, image5, image6, image7, image8];
let page = 1;
const overlay_buttons = [
{
icon: "trash",
color: "error",
invert_icon: true,
larger: false,
onclick: (event) => {
event.preventDefault();
event.stopPropagation();
},
},
{
label: "Download image",
color: "gray-lighter",
larger: false,
},
];
const new_image = () => {
const form = [
{
type: "file",
label: "Add Image",
id: "image",
},
{
type: "submit",
label: "Upload Image",
onsubmit: async (event) => {
const loading = event.currentTarget.id;
const data = await getFormData(form);
console.log(data);
showLoading(loading);
setTimeout(() => {
hideLoading(loading);
}, 2000);
},
},
];
showModal("Add Image", { form });
};
</script>
<GalleryCard>
<Gallery
title="Images"
pagination={true}
bind:page
{images}
grid="2x2"
new_image={() => new_image()}
{overlay_buttons}
/>
</GalleryCard>